TeleOp
This section will be explaining manual robot control (Teleop), which lasts 2 minutes and 15 seconds of the game. This documentation will be referring to the manual drive class, and specific parts of the RobotContainer class. More information about RobotContainer can be found here.
Driving
There are 2 main types of driving: field-oriented and robot-oriented.
Field-oriented driving involves a drive system that provides strafing motion in all directions and rotations with respect to the field. For example, if the robot is facing east and the joystick is pushed forward, the robot would move north.
Compared to robot-oriented driving, the joystick’s movement would be relative to the robot instead of the field. For example, if the robot was facing east and the joystick is pushed forward, the robot would move east.
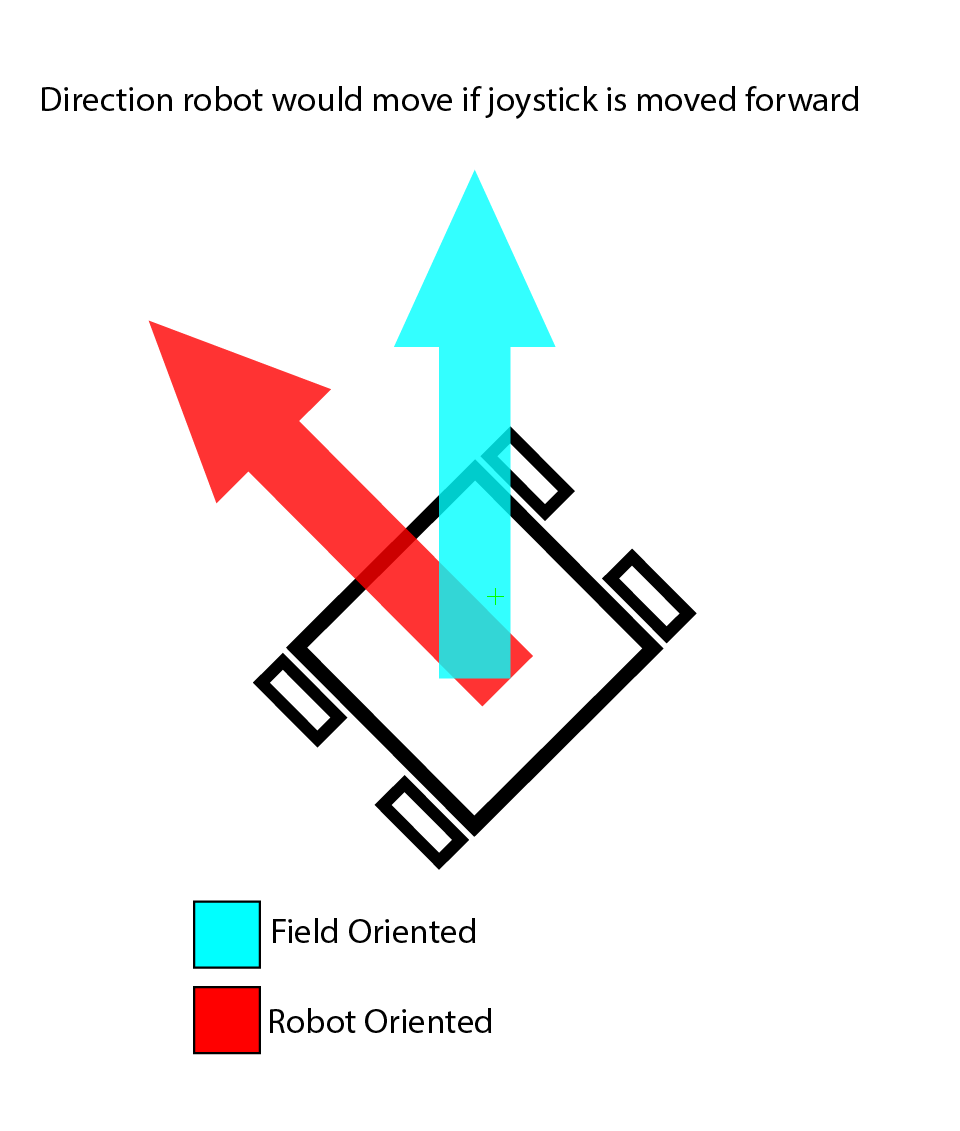
Field-oriented |
Robot-oriented |
|
---|---|---|
Requires IMU |
Yes (IMU drift*) |
No |
Joystick movement |
Relative to field |
Relative to robot |
* IMU drift may cause slight inaccuracy in field-oriented driving.
Here’s a demonstration of field-oriented control:
Drive Command
Since we will be using command based programming, a drive command has to be created to manually operate the robot.
Constructor
1private final Swerve mSwerve;
2private final XboxController mController;
3
4public ManualDrive(Swerve drive, XboxController controller) {
5 mSwerve = drive;
6 mController = controller;
7
8 // Adds the Swerve subsystem as a requirement to the command
9 addRequirements(mSwerve);
10}
Parameters:
drive
- a swerve object for the drivecontroller
- a XboxController method for the controller
A drive command has to be created to manually operate the robot. Calling the constructor will create the drive command, where it will then be used in RobotContainer.
execute
The execute method is called repeatedly when the command is scheduled. This is where the code for teleop will be written.
1@Override
2public void execute() {
3 // Drives with XSpeed, YSpeed, zSpeed
4 // True/false for field-oriented driving
5 mSwerve.drive(mController.getLeftY(), mController.getLeftX(), mController.getRightX(), true);
6}
Calling the Drive Command
Finally, the drive command has to be called in RobotContainer.
1// Create new instance of ManualDrive, passing Swerve and Controller as parameters
2private final ManualDrive mManualDriveCommand = new ManualDrive(mSwerve, mController);
3
4public RobotContainer() {
5 // Configure the button bindings
6 configureButtonBindings();
7
8 // set ManualDrive to be executed in teleop
9 mSwerve.setDefaultCommand(mManualDriveCommand);
10}
A new instance of ManualDrive is created, which also creates the drive command. To use the drive command in RobotContainer, the default command of the Swerve object is set to the manual drive object. When Teleop starts, the ManualDrive command will be automatically executed.
Note
Check out the code we use in these docs on our Github!